- You can locate a workbook on your computer and simply double-click it to open it, but you can also open a workbook from within the Excel program. Click the File tab.
- Sometimes we may want to Activate Workbook Or Worksheet using Excel VBA.You can use Activate method in VBA to Select the required Workbook Or WorkSheet using VBA and perform required tasks. For example, we may have 5 worksheets, we have to do some tasks in Sheet3.
Workbook Vs Worksheet Level Named Ranges: When you create a workbook level named range, there could only be one range with that name.So if you refer to it from external workbooks or worksheets in the same workbook, you don’t need to specify the worksheet name (as it’s available for use in the entire workbook). Create a workbook in Excel Excel makes it easy to crunch numbers. With Excel, you can streamline data entry with AutoFill. Then, get chart recommendations based on your data, and create them with one click. When you open Excel, you’ll see a window asking what you want to do: To open a blank workbook,double-click Blank workbook, and you’re good to go. That’s all there is to it. If you already have a workbook open, click the File tab from the left side of the Ribbon, then select New from the left sidebar.
This article provides code samples that show how to perform common tasks with workbooks using the Excel JavaScript API. For the complete list of properties and methods that the Workbook
object supports, see Workbook Object (JavaScript API for Excel). This article also covers workbook-level actions performed through the Application object.
The Workbook object is the entry point for your add-in to interact with Excel. It maintains collections of worksheets, tables, PivotTables, and more, through which Excel data is accessed and changed. The WorksheetCollection object gives your add-in access to all the workbook's data through individual worksheets. Specifically, it lets your add-in add worksheets, navigate among them, and assign handlers to worksheet events. The article Work with worksheets using the Excel JavaScript API describes how to access and edit worksheets.
Get the active cell or selected range
The Workbook object contains two methods that get a range of cells the user or add-in has selected: getActiveCell()
and getSelectedRange()
. getActiveCell()
gets the active cell from the workbook as a Range object. The following example shows a call to getActiveCell()
, followed by the cell's address being printed to the console.
The getSelectedRange()
method returns the currently selected single range. If multiple ranges are selected, an InvalidSelection error is thrown. The following example shows a call to getSelectedRange()
that then sets the range's fill color to yellow.
Create a workbook
Your add-in can create a new workbook, separate from the Excel instance in which the add-in is currently running. The Excel object has the createWorkbook
method for this purpose. When this method is called, the new workbook is immediately opened and displayed in a new instance of Excel. Your add-in remains open and running with the previous workbook.
The createWorkbook
method can also create a copy of an existing workbook. The method accepts a base64-encoded string representation of an .xlsx file as an optional parameter. The resulting workbook will be a copy of that file, assuming the string argument is a valid .xlsx file.
You can get your add-in's current workbook as a base64-encoded string by using file slicing. The FileReader class can be used to convert a file into the required base64-encoded string, as demonstrated in the following example.
Insert a copy of an existing workbook into the current one (preview)
Note
The Workbook.insertWorksheetsFromBase64
method is currently only available in public preview. To use this feature, you must use the preview version of the Office JavaScript API library from the Office.js CDN. The type definition file for TypeScript compilation and IntelliSense is found at the CDN and DefinitelyTyped. You can install these types with npm install --save-dev @types/office-js-preview
.For more information on our upcoming APIs, please visit Excel JavaScript API requirement sets.
The previous example shows a new workbook being created from an existing workbook. You can also copy some or all of an existing workbook into the one currently associated with your add-in. A Workbook has the insertWorksheetsFromBase64
method to insert copies of the target workbook's worksheets into itself. The other workbook's file is passed as a base64-encoded string, just like the Excel.createWorkbook
call.
The following example inserts another workbook into the current workbook. The new worksheets are inserted after the active worksheet. Note that []
is passed as the parameter for the InsertWorksheetOptionssheetNamesToInsert
property. This means that all the worksheets from the existing workbook are inserted into the current workbook.
Important
The insertWorksheetsFromBase64
method is supported for Excel on Windows, Mac, and the web. It's not supported for iOS. Additionally, in Excel on the web this method doesn't support source worksheets with PivotTable, Chart, Comment, or Slicer elements. If those objects are present, the insertWorksheetsFromBase64
method returns the UnsupportedFeature
error in Excel on the web.
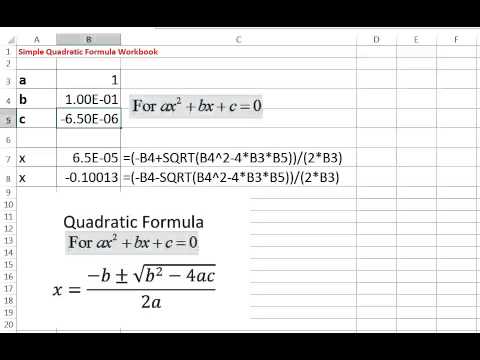
Protect the workbook's structure
Your add-in can control a user's ability to edit the workbook's structure. The Workbook object's protection
property is a WorkbookProtection object with a protect()
method. The following example shows a basic scenario toggling the protection of the workbook's structure.
The protect
method accepts an optional string parameter. This string represents the password needed for a user to bypass protection and change the workbook's structure.
Protection can also be set at the worksheet level to prevent unwanted data editing. For more information, see the Data protection section of the Work with worksheets using the Excel JavaScript API article.
Note
For more information about workbook protection in Excel, see the Protect a workbook article.
Access document properties
Workbook objects have access to the Office file metadata, which is known as the document properties. The Workbook object's properties
property is a DocumentProperties object containing these metadata values. The following example shows how to set the author
property.
Custom properties
You can also define custom properties. The DocumentProperties object contains a custom
property that represents a collection of key-value pairs for user-defined properties. The following example shows how to create a custom property named Introduction with the value 'Hello', then retrieve it.
Worksheet-level custom properties
Custom properties can also be set at the worksheet level. These are similar to document-level custom properties, except that the same key can be repeated across different worksheets. The following example shows how to create a custom property named WorksheetGroup with the value 'Alpha' on the current worksheet, then retrieve it.
Access document settings
A workbook's settings are similar to the collection of custom properties. The difference is settings are unique to a single Excel file and add-in pairing, whereas properties are solely connected to the file. The following example shows how to create and access a setting.
Access application culture settings

A workbook has language and culture settings that affect how certain data is displayed. These settings can help localize data when your add-in's users are sharing workbooks across different languages and cultures. Your add-in can use string parsing to localize the format of numbers, dates, and times based on the system culture settings so that each user sees data in their own culture's format.
Application.cultureInfo
defines the system culture settings as a CultureInfo object. This contains settings like the numerical decimal separator or the date format.
Some culture settings can be changed through the Excel UI. The system settings are preserved in the CultureInfo
object. Any local changes are kept as Application-level properties, such as Application.decimalSeparator
.
The following sample changes the decimal separator character of a numerical string from a ',' to the character used by the system settings.
Add custom XML data to the workbook
Excel's Open XML .xlsx file format lets your add-in embed custom XML data in the workbook. This data persists with the workbook, independent of the add-in.
A workbook contains a CustomXmlPartCollection, which is a list of CustomXmlParts. These give access to the XML strings and a corresponding unique ID. By storing these IDs as settings, your add-in can maintain the keys to its XML parts between sessions.
The following samples show how to use custom XML parts. The first code block demonstrates how to embed XML data in the document. It stores a list of reviewers, then uses the workbook's settings to save the XML's id
for future retrieval. The second block shows how to access that XML later. The 'ContosoReviewXmlPartId' setting is loaded and passed to the workbook's customXmlParts
. The XML data is then printed to the console.
Note
CustomXMLPart.namespaceUri
is only populated if the top-level custom XML element contains the xmlns
attribute.
Control calculation behavior
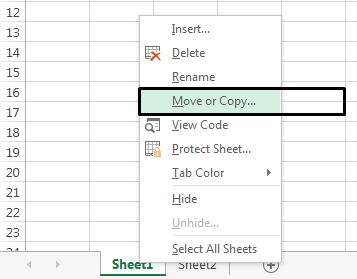
Set calculation mode
By default, Excel recalculates formula results whenever a referenced cell is changed. Your add-in's performance may benefit from adjusting this calculation behavior. The Application object has a calculationMode
property of type CalculationMode
. It can be set to the following values:
automatic
: The default recalculation behavior where Excel calculates new formula results every time the relevant data is changed.automaticExceptTables
: Same asautomatic
, except any changes made to values in tables are ignored.manual
: Calculations only occur when the user or add-in requests them.
Set calculation type
The Application object provides a method to force an immediate recalculation. Application.calculate(calculationType)
starts a manual recalculation based on the specified calculationType
. The following values can be specified:
full
: Recalculate all formulas in all open workbooks, regardless of whether they have changed since the last recalculation.fullRebuild
: Check dependent formulas, and then recalculate all formulas in all open workbooks, regardless of whether they have changed since the last recalculation.recalculate
: Recalculate formulas that have changed (or been programmatically marked for recalculation) since the last calculation, and formulas dependent on them, in all active workbooks.
Note
For more information about recalculation, see the Change formula recalculation, iteration, or precision article.
Temporarily suspend calculations
The Excel API also lets add-ins turn off calculations until RequestContext.sync()
is called. This is done with suspendApiCalculationUntilNextSync()
. Use this method when your add-in is editing large ranges without needing to access the data between edits.
Save the workbook

Workbook.save
saves the workbook to persistent storage. The save
method takes a single, optional saveBehavior
parameter that can be one of the following values:
Excel.SaveBehavior.save
(default): The file is saved without prompting the user to specify file name and save location. If the file has not been saved previously, it's saved to the default location. If the file has been saved previously, it's saved to the same location.Excel.SaveBehavior.prompt
: If file has not been saved previously, the user will be prompted to specify file name and save location. If the file has been saved previously, it will be saved to the same location and the user will not be prompted.
Caution
If the user is prompted to save and cancels the operation, save
throws an exception.
Close the workbook
Workbook.close
closes the workbook, along with add-ins that are associated with the workbook (the Excel application remains open). The close
method takes a single, optional closeBehavior
parameter that can be one of the following values:
Excel.CloseBehavior.save
(default): The file is saved before closing. If the file has not been saved previously, the user will be prompted to specify file name and save location.Excel.CloseBehavior.skipSave
: The file is immediately closed, without saving. Any unsaved changes will be lost.
See also
Protect Workbook In Excel
How to open Excel workbooks in new windows (open multiple windows)?
In this tutorial, we will show you how to open Excel workbooks in different windows steps by steps.
Open Excel workbooks in new windows with holding Shift key and clicking in Taskbar
For the usage of lowest versions of Excel, with holding the Shift key and clicking the Excel icon in the Taskbar, you can open Excel workbooks in multiple windows.
1. Firstly you need to open a workbook in advance, and then hold the Shift key on the keyboard.
2. Click the Excel icon in the Taskbar. See screenshot:
Then a new blank workbook is opened. If you need to edit the new opened workbook, just edit and save it. Otherwise, you can open another workbook you have created before in this new workbook window.
3. Click File (Office button) > Open from your new created workbook just now, In the Open dialog box, find and select the workbook and then click the Open button.
Then you can see the workbooks are opened in two separate Excel windows. You can repeat the above steps to open more Excel windows.
Open Excel workbooks in new windows with Start menu
Besides the above method, you can open Excel workbooks in new windows with Start menu. Please do as follows.
1. Click the Start button to show the menu. Type Excel in the Search box, then click Microsoft Excel in the Programs section. See screenshot:
2. When a new blank workbook opened, please click File (Office button) > Open, In the Open dialog box, find and select the workbook you want to open and then click the Open button.
3. To open multiple workbooks you want, you just need to repeat the above steps one by one.
Open Excel workbooks in new windows with creating shortcut
The last method for opening Excel workbooks in new window is to create a shortcut. Please do as follows.
1. Open the Office folder in your computer with following paths.
In Excel 2010: C:Program FilesMicrosoft OfficeOffice 14
In Excel 2007: C:Program FilesMicrosoft OfficeOffice12
2. In the opened Office folder, find the EXCEL icon, right click it and then select Create shortcut from the right-clicking menu. See screenshot:
3. Then a Shortcut prompt box pops up, click the Yes button.
4. Then an Excel shortcut is placed on the desktop. Double click it, and a new Excel window will be opened. Then you can click File (Office button) > Open to open the workbook that you need.
5. If you need to open multiple workbooks in new windows, you should repeat step 4 to open them one by one.
Note: For Excel 2013, the workbooks are opened in different windows by default.
Open Excel workbooks in new windows with Office Tab
In this section, I will show you a handy applications – Office Tab. With the Open In New Window feature of Office Tab, open workbooks in new windows is not a problem anymore.
1. After installing Office Tab, open the workbooks you want. Then you will see all workbooks are opened in an Excel window as tabbed browsing status.

2. If you want to open a specific workbook in a new window, please right click this workbook tab, and then click Open In New Window in the list. See screenshot:
Then the selected workbook is opened in a new window as below screenshot shown:
Tip.If you want to have a free trial of this utility, please go to Click for free trial of Office Tab first, and then go to apply the operation according above steps.
The Best Office Productivity Tools
Kutools for Excel Solves Most of Your Problems, and Increases Your Productivity by 80%
- Reuse: Quickly insert complex formulas, charts and anything that you have used before; Encrypt Cells with password; Create Mailing List and send emails...
- Super Formula Bar (easily edit multiple lines of text and formula); Reading Layout (easily read and edit large numbers of cells); Paste to Filtered Range...
- Merge Cells/Rows/Columns without losing Data; Split Cells Content; Combine Duplicate Rows/Columns... Prevent Duplicate Cells; Compare Ranges...
- Select Duplicate or Unique Rows; Select Blank Rows (all cells are empty); Super Find and Fuzzy Find in Many Workbooks; Random Select...
- Exact Copy Multiple Cells without changing formula reference; Auto Create References to Multiple Sheets; Insert Bullets, Check Boxes and more...
- Extract Text, Add Text, Remove by Position, Remove Space; Create and Print Paging Subtotals; Convert Between Cells Content and Comments...
- Super Filter (save and apply filter schemes to other sheets); Advanced Sort by month/week/day, frequency and more; Special Filter by bold, italic...
- Combine Workbooks and WorkSheets; Merge Tables based on key columns; Split Data into Multiple Sheets; Batch Convert xls, xlsx and PDF...
- More than 300 powerful features. Supports Office/Excel 2007-2019 and 365. Supports all languages. Easy deploying in your enterprise or organization. Full features 30-day free trial. 60-day money back guarantee.
Excel For Dummies Free
Office Tab Brings Tabbed interface to Office, and Make Your Work Much Easier
- Enable tabbed editing and reading in Word, Excel, PowerPoint, Publisher, Access, Visio and Project.
- Open and create multiple documents in new tabs of the same window, rather than in new windows.
- Increases your productivity by 50%, and reduces hundreds of mouse clicks for you every day!
or post as a guest, but your post won't be published automatically.
- To post as a guest, your comment is unpublished.if you do send to desktop you can avoid the pop-up asking you to put the shortcut on the desktop
- To post as a guest, your comment is unpublished.Thanks for your information guys, very very usefull
